Add New Tickets
In this section, you'll learn to:
- Capture data entered in Form.
- Add new tickets to the database.
Build UI
You need to build a Form to capture details for new tickets.
- On the Tickets page, drop a Modal widget on the canvas.
- On the top of the property pane, change the name from Modal1 to
mdlNewTicket
. - Select Modal Title. Change the title in the Text property to
New Ticket
. - Delete the default Close and Confirm buttons.
- Increase the width of the Modal using the resize handle.
- On the top of the property pane, change the name from Modal1 to
If you accidentally close the Modal, you can open it again by selecting the name of the modal mdlNewTicket under the Explorer tab in the Widgets section.
From under the Widgets tab, drop a Form widget inside the Modal. Delete the default Form title. Increase the width of the Form using the resize handle.
Drop two Input and three Select widgets inside the Form and set their properties as follows:
Properties - Input1- Widget Name:
c_user
- Data type:
Email
- Text:
User Email
- Required:
true
Properties - Input2- Widget Name:
c_description
- Data type:
Multi-line text
- Text:
Description
- Required:
true
Properties - Select1- Widget Name:
c_category
- Options:
[
{
"label": "Billing",
"value": "Billing"
},
{
"label": "Account",
"value": "Account"
},
{
"label": "Technical",
"value": "Technical"
}
]- Default selected value: empty
- Label:
Category
Properties - Select2- Widget Name:
c_assignee
- Options:
[
{
"label": "John Doe",
"value": "John Doe"
},
{
"label": "Karmila Fox",
"value": "Karmila Fox"
},
{
"label": "Sarah Smith",
"value": "Sarah Smith"
}
]- Default selected value: empty
- Label:
Assignee
Properties - Select3- Widget Name:
c_priority
- Options:
[
{
"label": "High",
"value": "high"
},
{
"label": "Medium",
"value": "medium"
},
{
"label": "Low",
"value": "low"
}
]- Default selected value: empty
- Label:
Priority
The output should look something like this:
- Widget Name:
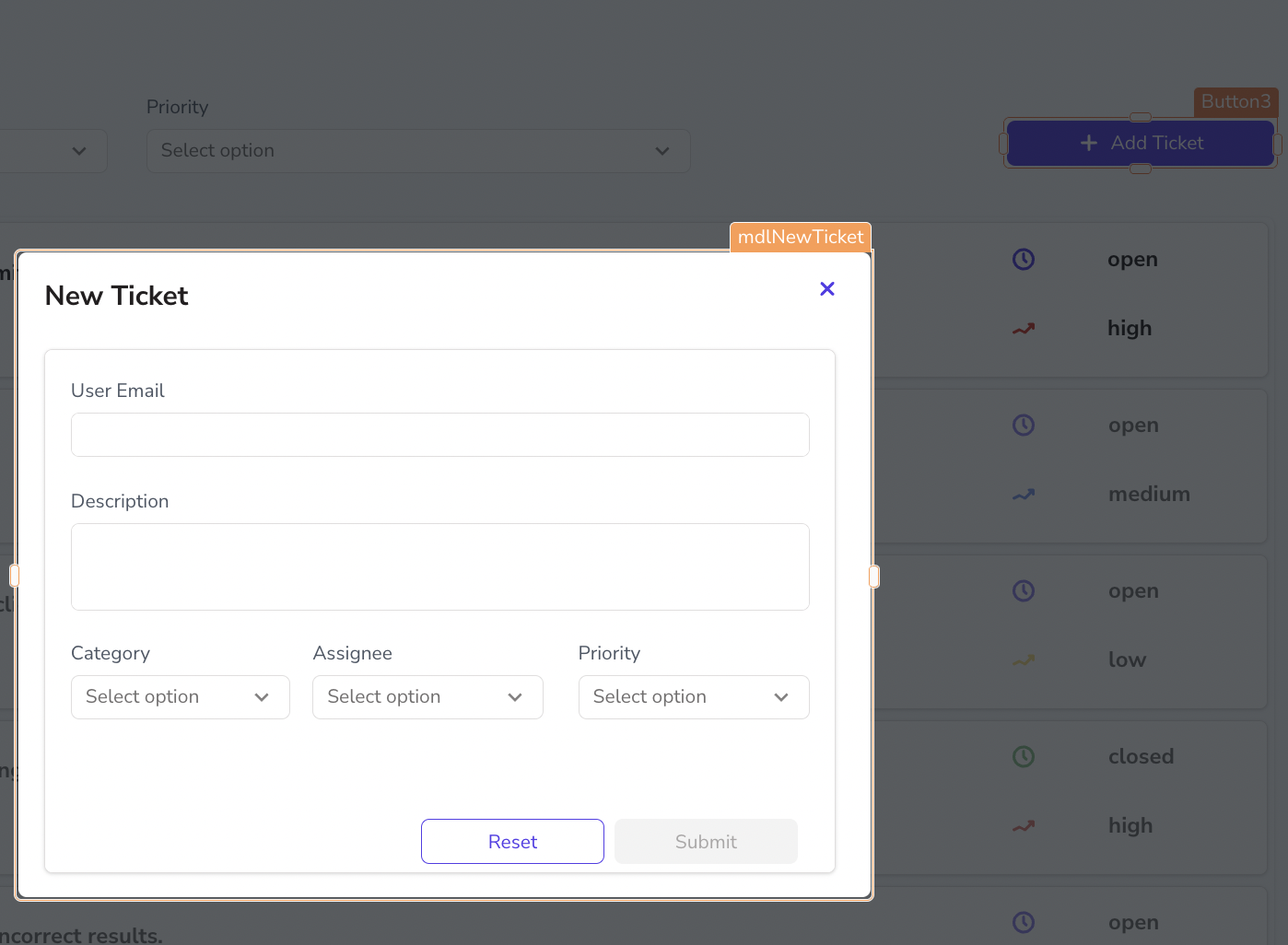
Create add ticket query
You have to create an insert query to add the data entered in the Form into the database.
Select the Explorer tab on the Entity Explorer to the screen's left.
Click the + icon next to Queries/JS.
Select supportTickets query from the list of options.
Rename the query to
createTicket
. Click the white space near the query templates.Write the following SQL query.
INSERT INTO support_ticket ("created_at", "user", "description", "status", "priority", "category", "assigned_to")
VALUES ('{{moment().format("LLL")}}', '{{c_user.text}}', '{{c_description.text}}', 'open', '{{c_priority.selectedOptionValue}}', '{{c_category.selectedOptionValue}}', '{{c_assignee.selectedOptionValue}}');Go back to the canvas by clicking on the ← Back button above the query editor.
Submit new tickets
On the Entity Explorer, under JS Objects section, click on utils.
You have to write a new function in the utils JS Object to call the createTicket insert query. Post running the insert query, we want to refresh the List to show the newly added ticket and then close the Modal. At the end of the getFilteredTickets function that you wrote earlier, add a comma
,
on line 24 as shown in Fig 2. Write a new function as shown below:
createTicket: async () => {
await createTicket.run()
.then(()=> this.getFilteredTickets())
.then(()=> closeModal('mdlNewTicket'))
}
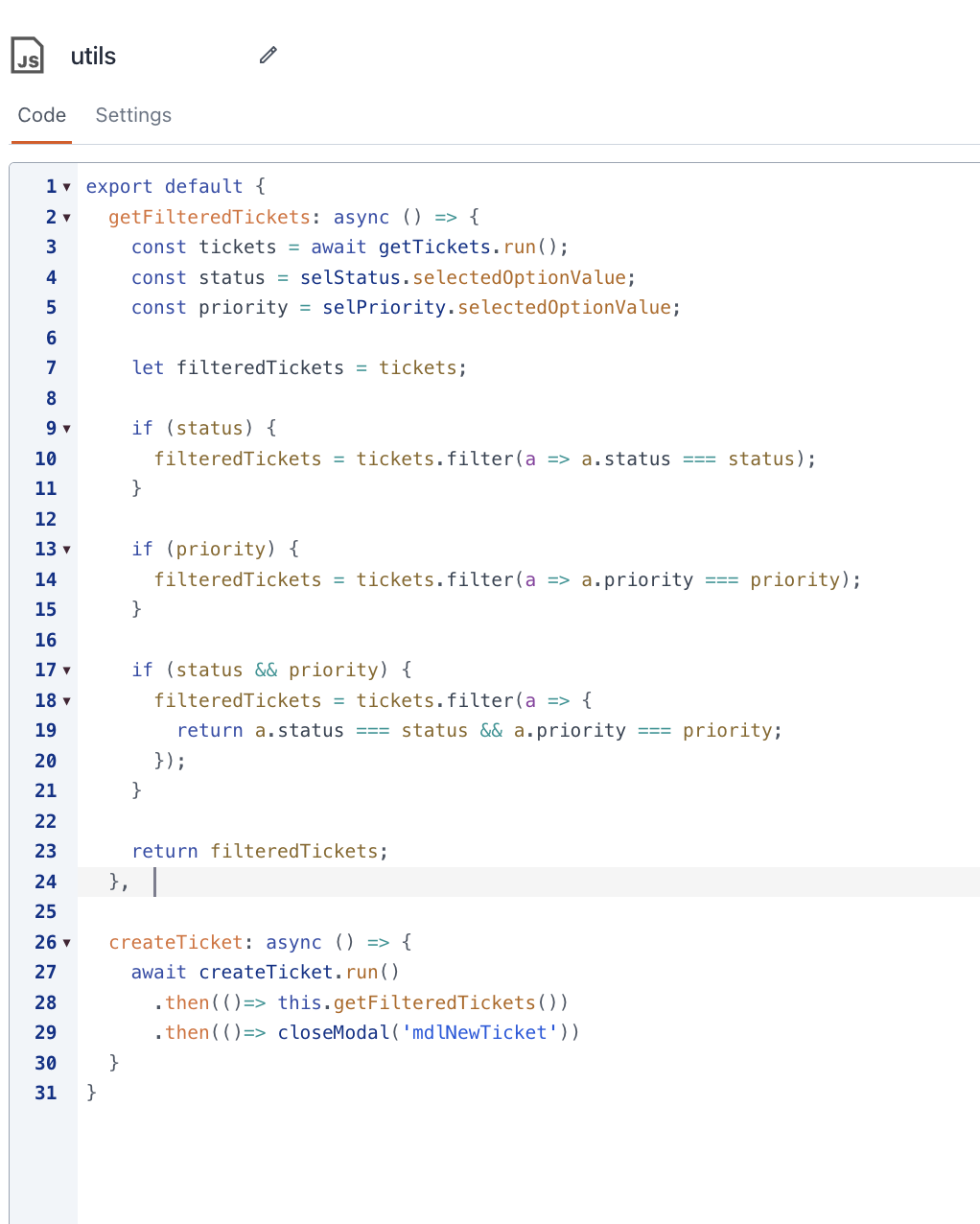
Go back to the canvas by clicking on the Tickets page. Drop a Button widget on the canvas to the top right of the screen above the lstTicketDetails List.
- Change the Label property to
Add Ticket
. - Click the + icon next to the onClick event.
- In the Select an action list, select Show Modal.
- In the Modal name list, select the mdlNewTicket modal.
- Click the Style tab, in the Select icon list, search
plus
and select the icon.
- Change the Label property to
Click the Add Ticket button to open the Modal.
Select the Submit button in the Form.
- Click the + icon next to the onClick event.
- In the Select an action list, select Execute a JS function > utils > createTicket.
Enter test data in the Form and click the Submit button to verify that the new ticket details are added in the database and on the List.